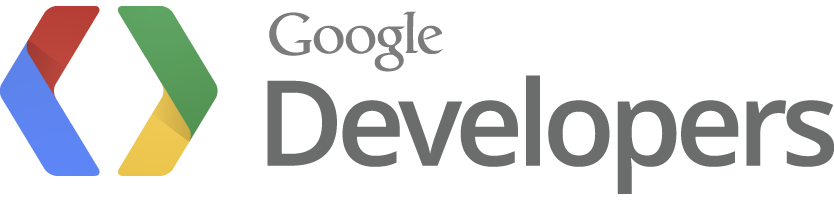
Step 1: Get the Google App Engine SDK
Step 2: Write code
Step 3: Deploy and test locally
Step 4: Deploy to App Engine
Always remember: developers.google.com/appengine
How does a link shortener work?!
The alogrithm
avian-silo-347.appspot.com
lnkr.co.za
is easier to remember, so I mapped the domainsversion.module.applicationID.appspot.com
deferred.defer(utils.updateMetric, 'create', os.getenv('HTTP_X_APPENGINE_COUNTRY'))
@endpoints.api(name='shortlink',version='v1', description='Short Link API') class ShortLinkApi(remote.Service): @endpoints.method(CreateShortLinkMessageRequest, CreateShortLinkMessageResponse, name='create', path= '/create', http_method = 'POST') def createShortLink(self, request): link = request.short_link
/_ah/api/shortlink/v1/create
class ShortLink(ndb.Model): """Models a short URL: short link, target, date created.""" short_link = ndb.StringProperty() created_date = ndb.IntegerProperty() target_link = ndb.StringProperty() class MetricCounter(ndb.Model): """A metric.""" name = ndb.StringProperty() country = ndb.StringProperty() value = ndb.IntegerProperty() day = ndb.IntegerProperty()
Write to Datastore
link = ShortLink(parent = pk, short_link = sl, target_link = request.target_link, created_date = long(time.time()*1000)) link.put()
Transactions
@ndb.transactional def incrementCounter(key): obj = key.get() obj.value += 1 obj.put()
Step 1: Load the library for the API
<script src="//apis.google.com/js/client.js?onload=init"></script> <script> var ROOT = ''; if (window.location.host.indexOf('localhost') == 0) { ROOT = 'http://' + window.location.host + '/_ah/api'; } else { ROOT = 'https://'+hostname+'/_ah/api'; } gapi.client.load('shortlink', 'v1', function() { console.log('Loaded shortlink library'); }, ROOT);
Step 2: Call the API
var inObj = {'target_link': 'http://www.danielacton.com'}; gapi.client.shortlink.create(inObj).execute(function(resp) { // Do something with the response }); </script>
Using WebStorage
Specific to browser
if (window.localStorage) { var currentLinks = storage.getItem('shortlinks'); if (currentLinks) { // Do something with currentLinks } storage.setItem('shortlinks', currentLinks); }
Use-case: consistent colours
$dark-blue: #4884B2; color: $dark-blue;
Use-case: vendor prefixes
@mixin box-shadow() { -moz-box-shadow: 2px 2px 2px 2px $mid-grey; -webkit-box-shadow: 2px 2px 2px 2px $mid-grey; box-shadow: 2px 2px 2px 2px $mid-grey; } @include box-shadow();
Vendor prefixes omitted for brevity
#someElement { transition: opacity 1s ease-in-out; }
background: linear-gradient(top, $white, $mid-grey);
.grid-row:nth-child(even) { background: $white; }
@media all and (max-width: 420px) { .grid-caption { width: 360px; } }
<a href="https://plus.google.com/share?url=http://lnkr.co.za/aa13"
onclick="javascript:windowOpenText;">
<img src="https://www.gstatic.com/images/icons/gplus-16.png"
alt="Share on Google+"/>Share on</a>
function drawVisualization() { var data = google.visualization.arrayToDataTable([ ["country", "count"], ["ZA", 22], ["NG", 1], ["NL", 1], ["GB", 5], ["KE", 1], ["FR", 3] ]); var geochart = new google.visualization.GeoChart(document.querySelector('#viz-use')); geochart.draw(data, {width: 556, height: 347}); };
$ appengine-sdk-directory/endpointscfg.py \ get_client_lib java [--hostname localhost] \ your_module.YourServiceClass
If hostname not specified
@endpoints.api( ... hostname='your_app_id.appspot.com')
application: your_app_id version: 1 runtime: python27
This will give you a zip file with all required libraries
Shortlink service = new Shortlink.Builder( AndroidHttp.newCompatibleTransport(), new GsonFactory(), null); service.setRootUrl("https://your_app_id.appspot.com/_ah/api/"); service.setServicePath("shortlink/v1/"); service.build();
List Short links
service.list().execute().getShortLinks()
Create a short link
ApiMessagesCreateShortLinkMessageRequest request = new ApiMessagesCreateShortLinkMessageRequest(); request.setTargetLink(targetUrl); request.setShortLink(shortLink); ApiMessagesCreateShortLinkMessageResponse response = service.create(request).execute();
A conundrum!
private class ListShortLinks extends AsyncTask{ protected String doInBackground(String... params) { // Invoke the Shortlink API return aStringResult; } protected void onPostExecute(String result) { // Do something with the string result } protected void onPreExecute() {} protected void onProgressUpdate(Void... values) {} } // In your Activity new ListShortLinks().execute("");