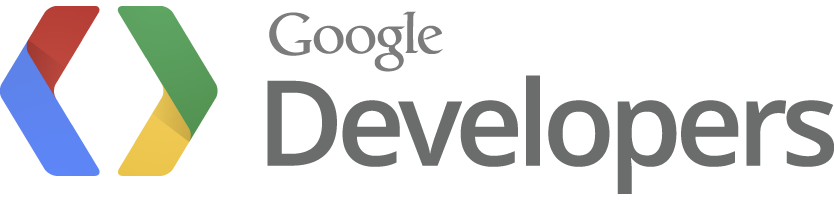
Step 1: Get the Google App Engine SDK
Step 2: Write code
Step 3: Test locally
Step 4: Deploy to App Engine
Always remember: developers.google.com/appengine
First
Then
src/
war/
index.html
style/
js/
WEB-INF/
web.xml
- Servlet configurationappengine-web.xml
- App Engine configurationStep 1: make sure your web.xml
is updated
<welcome-file-list> <welcome-file>index.html</welcome-file> </welcome-file-list>
Step 2: Define your servlet(s)
<servlet> <servlet-name>Exercise2</servlet-name> <servlet-class>com.samples.exercises.Exercise2Servlet</servlet-class> </servlet>
Step 3: Map your servlet to a url
<servlet-mapping> <servlet-name>Exercise2</servlet-name> <url-pattern>/exercise2</url-pattern> </servlet-mapping>
Step 4: Write servlet code
public class Exercise2Servlet extends HttpServlet { public void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { resp.setContentType("text/html"); resp.getWriter().println("<p>This is another servlet</p>"); } }
javax.servlet.http.HttpServlet
doGet(...)
method (or doPost
, etc.)
web.xml
/exercise2
in web.xml
DatastoreService datastore = DatastoreServiceFactory.getDatastoreService(); // Kind is Person, Identifier is danacton Entity dan = new Entity("Person", "danacton"); dan.setProperty("name", "Dan"); dan.setProperty("hairColour", "brown"); datastore.put(dan); Entity danChild = new Entity("Person", "danchild1", dan.getKey()); danChild.setProperty("name", "Dan Child"); danChild.setProperty("hairColour", "brown"); datastore.put(danChild);
Visit http://localhost:8888/_ah/admin
Update
Entity dan = datastore.get(KeyFactory.stringToKey(...)); dan.setProperty("name", "Daniel"); datastore.put(dan); // key from datastore viewer for now
Delete
datastore.delete(KeyFactory.stringToKey(...)); // key from datastore viewer for now
Filter nameFilter = new FilterPredicate("hairColour", FilterOperator.EQUAL, "brown"); Query q = new Query("Person").setFilter(nameFilter); resp.setContentType("text/html"); PreparedQuery pq = datastore.prepare(q); for (Entity result : pq.asIterable()) { String name = (String) result.getProperty("name"); resp.getWriter().println("Person <b>" + name + "</b> has brown hair<br/>"); }
@PersistenceCapable public class Person { @PrimaryKey @Persistent(valueStrategy = IdGeneratorStrategy.IDENTITY) private Long id; @Persistent private String name; @Persistent private String hairColour; public Person() {} // Getters and setters }
src/META-INF/jdoconfig.xml
for configurationpackage com.samples.exercises.dataobjects; import javax.jdo.JDOHelper; import javax.jdo.PersistenceManagerFactory; public final class PMF { private static final PersistenceManagerFactory pmfInstance = JDOHelper.getPersistenceManagerFactory("transactions-optional"); private PMF() {} public static PersistenceManagerFactory get() { return pmfInstance; } }
PersistenceManager pm = PMF.get().getPersistenceManager(); Person dan = new Person(); dan.setName("Dan"); dan.setHairColour("brown"); Person danChild = new Person(); danChild.setName("Dan Child"); danChild.setHairColour("brown"); try { pm.makePersistent(dan); pm.makePersistent(danChild); } finally { pm.close(); }
Update
PersistenceManager pm = PMF.get().getPersistenceManager(); Person dan = pm.getObjectById(Person.class, ...); dan.setName("Daniel"); pm.makePersistent(dan); // id from datastore viewer for now
Delete
PersistenceManager pm = PMF.get().getPersistenceManager(); Person dan = pm.getObjectById(Person.class, ...); pm.deletePersistent(dan); // id from datastore viewer for now
PersistenceManager pm = PMF.get().getPersistenceManager(); Query q = pm.newQuery(Person.class, "hairColour == 'brown' order by name desc"); resp.setContentType("text/html"); List<Person> results = (List<Person>) q.execute(); for (Person p : results) { String name = p.getName(); resp.getWriter().println("Person <b>" + name + "</b> has brown hair<br/>"); }
war
directoryStart by denoting this is a JSP page
<%@page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
Import required classes
<%@page import="java.util.Date" %>
Add Java code to your JSP pages
<b><% out.println(new Date()); %></b>
<%@page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@page import="java.util.Date" %> <!DOCTYPE html> <html> <head> <title>A simple example</title> </head> <body> <p> The date is: <b><% out.println(new Date()); %></b> </p> </body> </html>
Before, we hard-coded keys. Now, we pass them around.
Using HTTP GET
http://localhost:8888/update?id=4
Using HTTP POST with a form
<form action="/update" method="POST"> <input type="hidden" name="id" value="4"> </form>
In your code
<% String val = "nothing"; if (request.getParameter("id") != null) { val = request.getParameter("id"); } %> The id you passed is <b><%=val%></b>