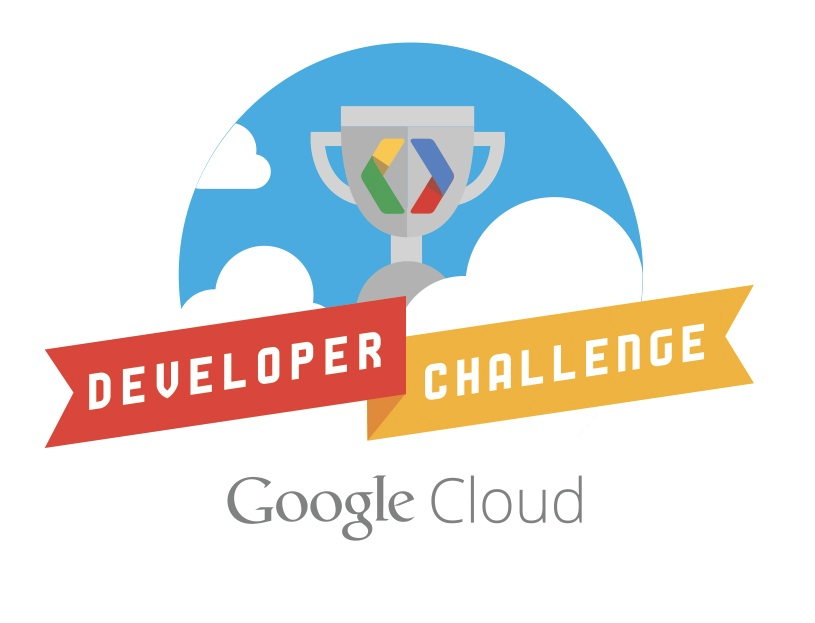
The evolution of HTML for rich web applications
HTML5 is more than just HTML
Organisations and Standards
All you need is <!DOCTYPE html>
These slides are HTML5
Examples of new semantic/structural elements
<section>
- indicates a section of the web page<article>
- indicates an independent piece of content <header>
- indicates header content - titles, etc.<footer>
- indicates content in the footer, e.g. copyright<nav>
- indicates navigational separationExamples of other new elements
<video>
- indicates a section of the web page<audio>
- indicates an independent piece of content <canvas>
- used to draw with JavascriptQuery Selectors
var elts = document.querySelectorAll('div'); var elt = document.querySelector('#elementId'); var elts = document.querySelectorAll('.elementClass'); var input = element.querySelector('input[type=file]');
<input list="newElementTypes" /> <datalist id="newElementTypes"> <option value="section"/> <option value="header"/> <option value="footer"/> <option value="progress"/> </datalist>
Example
Start typing, it should autocomplete
<progress value="0" id="progressBar" max="100"></progress>
document.querySelector('#progressBar').value += 10;
Example
<canvas id="canvas" width="320" height="240"></canvas>
var c = document.querySelector('#canvas'); var ctx = c.getContext('2d'); ctx.drawImage(someImage, 0, 0);
Example
To change the picture, load an image on slide 12
We saw datalist, progress and canvas
There are many more, like
<div class="entry" data-entryId="1234">
<input type="text|number|email|tel" />
<svg>...</svg>
if (navigator.geolocation) { navigator.geolocation.getCurrentPosition( successFunction, errorFunction, {timeout: 5000}); } else { console.log('Geolocation is not supported.'); }
Example
if (window.localStorage) { window.localStorage.setItem('key', 'value'); value = window.localStorage.getItem('key'); } else { console.log('Local storage is not supported.'); }
Example
if (window.FileReader) { var reader = new FileReader(); reader.readAsDataURL(document.getElementById('file').files[0]); reader.onload = function(event) { // do something with the content }; reader.onerror = function() { // let the user know something went wrong }; } else { console.log('File API is not supported.'); }
Example
We saw Geolocation, Local Storage and File API
There are many more, like
var socket = new WebSocket('ws://someUrl');
- connect to a WebSocket servervar db = window.openDatabase(name, version, description, size);
- create or open an sql-like database client-side<html manifest="mymanifest">
- instructs what to cacheWeb Fonts
@font-face { font-family: 'Droid Sans'; font-style: normal; font-weight: normal; src: local('Droid Sans'), url(url_to_ttf_file) format('truetype'); }
OR
@import url(http://fonts.googleapis.com/css?family=Cinzel+Decorative);
THEN
p { font-family: "Droid Sans", Arial, sans-serif; }
Rounded corners
.roundedButton { border-radius: 5px; -moz-border-radius: 5px; border: 1px solid #bbb; background: #c0c0c0; }
Transitions
#movingBox { -webkit-transition: all 1s ease-in-out; -moz-transition: all 1s ease-in-out; -o-transition: all 1s ease-in-out; background: red; height: 50px; width: 50px; }
document.querySelector('#movingBox').style.background = 'blue'; document.querySelector('#movingBox').style.width = '100px';
Transforms
#movingBox { -webkit-transition: all 1s ease-in-out; -moz-transition: all 1s ease-in-out; -o-transition: all 1s ease-in-out; background: red; height: 50px; width: 50px; }
document.querySelector('#movingBox').style['-moz-transform'] = 'rotateX(45deg)';
We saw Web Fonts, Rounded Corners, Transitions and Transforms
There are many more, like
input[type="text"]
- select input elements with the attribute type set to texttr:nth-child(even)
- select even-numbered table rowsdiv:not(.someClass)
- select div elements with class other than someClasstext-shadow
and box-shadow
- drop shadow for text and boxesThe problem
You have some options
Adapting to the screen size
@media only screen and (min-width: 320px) and (max-width: 479px) { /* Screen is 320-480px wide */ } @media only screen and (min-width: 480px) { /* Screen is more than 480px wide */ }
You can apply style based on a number of criteria
min-resolution
orientation
min-device-pixel-ratio
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1">
Useful links: