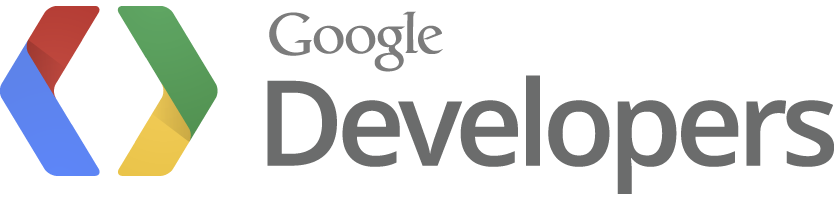
In App Engine 101 we covered
Here, we cover
java.util.logging
approachLogger log = Logger.getLogger(CreateServlet.class.getName()); log.info("A log message!");
Serializable
Make your class Serializable
public class Person implements Serializable
Use code to check and put in cache similar to this
String key = "Person"+id; MemcacheService syncCache = MemcacheServiceFactory.getMemcacheService(); syncCache.setErrorHandler(ErrorHandlers.getConsistentLogAndContinue(Level.INFO)); Person p = (Person) syncCache.get(key); if (p == null) { log.info("Cache miss for " + key); p = pm.getObjectById(Person.class, id); syncCache.put(key, p); } else { log.info("Cache hit for " + key); }
Blobstore is a storage service for arbitrary-size binary data
Step 1: Create a form in your JSP that posts to a special Blobstore URL
<%@ page import="com.google.appengine.api.blobstore.BlobstoreServiceFactory" %> <%@ page import="com.google.appengine.api.blobstore.BlobstoreService" %> <% BlobstoreService blobstoreService = BlobstoreServiceFactory.getBlobstoreService(); %> <form action="<%= blobstoreService.createUploadUrl("/uploadpicture") %>" method="post" enctype="multipart/form-data"> <input type="file" name="pictureFile"> </form>
Step 2: Create a servlet that gets passed the information once uploaded
BlobstoreService blobstoreService = BlobstoreServiceFactory.getBlobstoreService(); ImagesService imagesService = ImagesServiceFactory.getImagesService(); Map<String, List<BlobKey>> blobs = blobstoreService.getUploads(req); if (blobs.size() == 0) { resp.sendRedirect("/create.jsp"); } else { BlobKey k = blobs.get("pictureFile").get(0); // Do something with the blob key }
Step 3: Use the Image Service to create a serving URL and a thumbnail URL
ServingUrlOptions soOriginal = ServingUrlOptions.Builder.withBlobKey(k); ServingUrlOptions soThumb = ServingUrlOptions.Builder.withBlobKey(k).imageSize(100); String origUrl = imagesService.getServingUrl(soOriginal); String thumbUrl = imagesService.getServingUrl(soThumb); req.setAttribute("origUrl", origUrl); req.setAttribute("thumbUrl", thumbUrl); req.getRequestDispatcher("/create.jsp").forward(req, resp);
Step 4: Use the serving URL and thumbnail URL in your JSP page
<% if (request.getAttribute("thumbUrl") != null) { %> <img src="<%=request.getAttribute("thumbUrl")%>"> <% } %>
Google Cloud Endpoints can help!
Step 1: Create an endpoint class
Annotate with @Api
@Api(name = "person", version = "v1", description = "Person API") public class PersonEndpoint { }
Step 2: Create API Methods
Annotate with @ApiMethod
@ApiMethod(name = "read", httpMethod = HttpMethod.GET, path = "/person") public Person readPerson(@Named("id") Long id) { PersistenceManager pm = PMF.get().getPersistenceManager(); Person person = null; try { person = pm.getObjectById(Person.class, id); } finally { pm.close(); } return person; }
Step 3: Create the library
Step 4: Use the library
<script> function init() { var ROOT = '//' + window.location.host + '/_ah/api'; gapi.client.load('person', 'v1', function() { console.log('Loaded API'); }, ROOT); } </script> <script src="https://apis.google.com/js/client.js?onload=init"></script>
gapi.client.person.list().execute(com.samples.exercises.displayPeople); com.samples.exercises.displayPeople = function(datalist) { }
Some explanations ...
@Named
tells your method to use the "id" parameter@Nullable
tells your method the parameter is optional@Api(name = "person", version = "v1", description = "Person API") public class PersonEndpoint { @ApiMethod(name = "list", path = "/list", httpMethod = HttpMethod.GET) public CollectionResponselistPersons( @Nullable @Named("cursor") String cursorString, @Nullable @Named("limit") Integer limit) { } @ApiMethod(name = "create", httpMethod = HttpMethod.POST, path = "/person") public Person insertPerson(Person person) { } @ApiMethod(name = "remove", httpMethod = HttpMethod.DELETE, path = "/person") public void removePerson(@Named("id") Long id) { } @ApiMethod(name = "read", httpMethod = HttpMethod.GET, path = "/person") public Person readPerson(@Named("id") Long id) { } }
Signin options (specified when creating your app)
Specify this in your web.xml
<security-constraint> <web-resource-collection> <url-pattern>/*</url-pattern> </web-resource-collection> <auth-constraint> <role-name>*</role-name> </auth-constraint> </security-constraint>